- Python Slots Property For Sale
- Python Slots Property Management
- Python Slots Property Online
- Class Property Python
- Python Set Property
- Python Slots Property Search
Next Chapter: Implementing a Property Decorator
In Python, property is a built-in function that creates and returns a property object. The syntax of this function is: property (fget=None, fset=None, fdel=None, doc=None). Organized Robbery George Bernard Shaw once said 'Property is organized robbery.' This is true in a very positive sense in Python as well: A property in Python 'robs' the necessity to need getters and setters for attributes and to make it possible to start with public attributes instead of having everything private! We mentioned in the beginning that slots are preventing a waste of space with objects. Since Python 3.3 this advantage is not as impressive any more. With Python 3.3 Key-Sharing Dictionaries are used for the storage of objects.
Properties vs. Getters and Setters
It should store constants as class-level variables, and other values as instance-variables (or better yet properties). Then all of your methods should belong to that class. Keep related constants in an Enum. You have a bunch of constants related to what the slot reel is showing.
Python Slots Property For Sale
Properties
Getters(also known as 'accessors') and setters (aka. 'mutators') are used in many object oriented programming languages to ensure the principle of data encapsulation. Data encapsulation - as we have learnt in our introduction on Object Oriented Programming of our tutorial - is seen as the bundling of data with the methods that operate on them. These methods are of course the getter for retrieving the data and the setter for changing the data. According to this principle, the attributes of a class are made private to hide and protect them from the other codes.
Unfortunately, it is widespread belief that a proper Python class should encapsulate private attributes by using getters and setters. As soon as one of these programmers introduces a new attribute, he or she will make it a private variable and creates 'automatically' a getter and a setter for this attributes. Such programmers may even use an editor or an IDE, which automatically creates getters and setters for all private attributes. These tools even warn the programmer if she or he uses a public attribute! Java programmers will wrinkle their brows, screw up their noses, or even scream with horror when they read the following: The Pythonic way to introduce attributes is to make them public.
We will explain this later. First, we demonstrate in the following example, how we can design a class in a Javaesque way with getters and setters to encapsulate the private attribute self.__x
:
We can see in the following demo session how to work with this class and the methods:
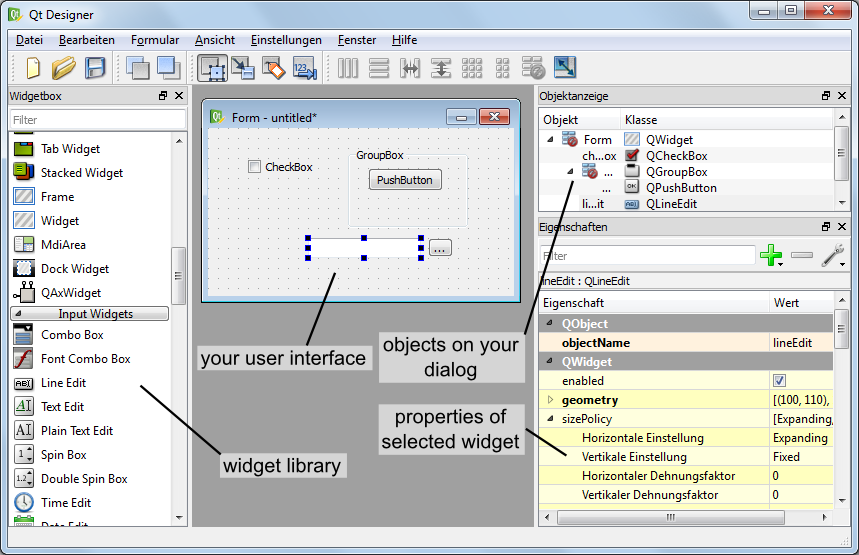

What do you think about the expression 'p1.set_x(p1.get_x()+p2.get_x())'? It's ugly, isn't it? It's a lot easier to write an expression like the following, if we had a public attribute x:
Python Slots Property Management
Such an assignment is easier to write and above all easier to read than the Javaesque expression.
Let's rewrite the class P in a Pythonic way. No getter, no setter and instead of the private attribute self.__x
we use a public one:
Beautiful, isn't it? Just three lines of code, if we don't count the blank line!
'But, but, but, but, but ... ', we can hear them howling and screaming, 'But there is NO data ENCAPSULATION!'Yes, in this case there is no data encapsulation. We don't need it in this case. The only thing get_x and set_x in our starting example did was 'getting the data through' without doing anything.
But what happens if we want to change the implementation in the future? This is a serious argument. Let's assume we want to change the implementation like this: The attribute x can have values between 0 and 1000. If a value larger than 1000 is assigned, x should be set to 1000. Correspondingly, x should be set to 0, if the value is less than 0.
It is easy to change our first P class to cover this problem. We change the set_x method accordingly:
The following Python session shows that it works the way we want it to work:
But there is a catch: Let's assume we designed our class with the public attribute and no methods. People have already used it a lot and they have written code like this:
Our new class means breaking the interface. The attribute x is not available anymore. That's why in Java e.g. people are recommended to use only private attributes with getters and setters, so that they can change the implementation without having to change the interface.
But Python offers a solution to this problem. The solution is called properties!
The class with a property looks like this:
A method which is used for getting a value is decorated with '@property', i.e. we put this line directly in front of the header. The method which has to function as the setter is decorated with '@x.setter'. If the function had been called 'f', we would have to decorate it with '@f.setter'.Two things are noteworthy: We just put the code line 'self.x = x' in the __init__
method and the property method x is used to check the limits of the values. The second interesting thing is that we wrote 'two' methods with the same name and a different number of parameters 'def x(self)' and 'def x(self,x)'. We have learned in a previous chapter of our course that this is not possible. It works here due to the decorating:
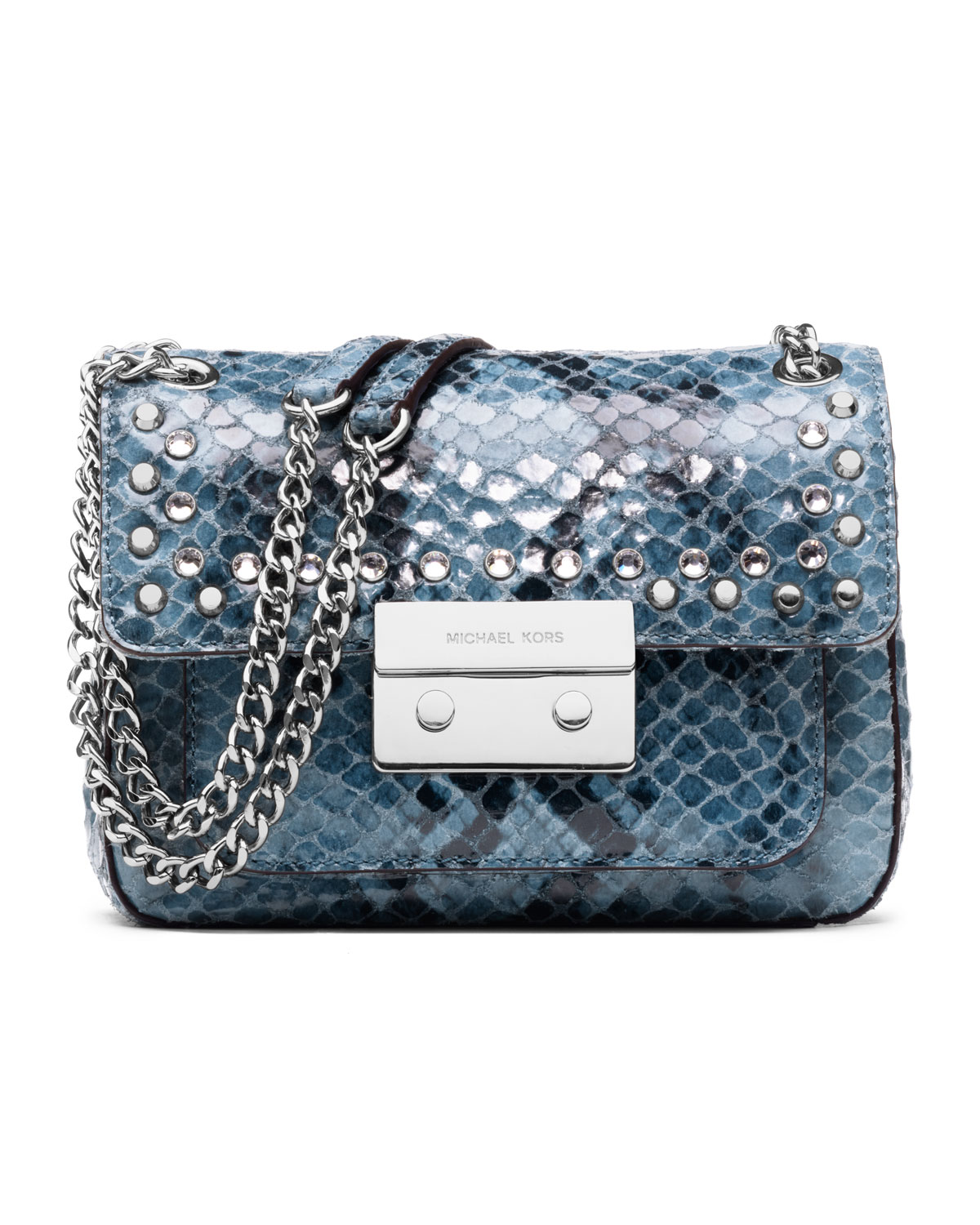
Alternatively, we could have used a different syntax without decorators to define the property. As you can see, the code is definitely less elegant and we have to make sure that we use the getter function in the __init__
method again:
There is still another problem in the most recent version. We have now two ways to access or change the value of x: Either by using 'p1.x = 42' or by 'p1.set_x(42)'. This way we are violating one of the fundamentals of Python: 'There should be one-- and preferably only one --obvious way to do it.' (see Zen of Python)
We can easily fix this problem by turning the getter and the setter methods into private methods, which can't be accessed anymore by the users of our class P:
Even though we fixed this problem by using a private getter and setter, the version with the decorator '@property' is the Pythonic way to do it!
Python Slots Property Online
From what we have written so far, and what can be seen in other books and tutorials as well, we could easily get the impression that there is a one-to-one connection between properties (or mutator methods) and the attributes, i.e. that each attribute has or should have its own property (or getter-setter-pair) and the other way around. Even in other object oriented languages than Python, it's usually not a good idea to implement a class like that. The main reason is that many attributes are only internally needed and creating interfaces for the user of the class increases unnecessarily the usability of the class. The possible user of a class shouldn't be 'drowned' with umpteen - of mainly unnecessary - methods or properties!
The following example shows a class, which has internal attributes, which can't be accessed from outside. These are the private attributes self.__potential
_physical
and self.__potential_psychic
. Furthermore we show that a property can be deduced from the values of more than one attribute. The property 'condition' of our example returns the condition of the robot in a descriptive string. The condition depends on the sum of the values of the psychic and the physical conditions of the robot.
Public instead of Private Attributes
Let's summarize the usage of private and public attributes, getters and setters, and properties: Let's assume that we are designing a new class and we pondering about an instance or class attribute 'OurAtt', which we need for the design of our class. We have to observe the following issues:
- Will the value of 'OurAtt' be needed by the possible users of our class?
- If not, we can or should make it a private attribute.
- If it has to be accessed, we make it accessible as a public attribute
- We will define it as a private attribute with the corresponding property, if and only if we have to do some checks or transformation of the data. (As an example, you can have a look again at our class P, where the attribute has to be in the interval between 0 and 1000, which is ensured by the property 'x')
- Alternatively, you could use a getter and a setter, but using a property is the Pythonic way to deal with it!
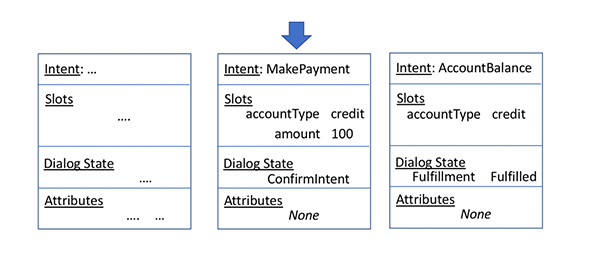
Let's assume we defined 'OurAtt' as a public attribute. Our class has been successfully used by other users for quite a while. Now comes the point which frightens some traditional OOPistas out of their wits: Imagine 'OurAtt' has been used as an integer. Now, our class has to ensure that 'OurAtt' has to be a value between 0 and 1000? Without property, this is really a horrible scenario! Due to properties it's easy: We create a property version of 'OurAtt'.
This is great, isn't it? You can start with the simplest implementation imaginable, and you are free to later migrate to a property version without having to change the interface! So properties are not just a replacement for getters and setters!
Something else you might have already noticed: For the users of a class, properties are syntactically identical to ordinary attributes.
Next Chapter: Implementing a Property Decorator
The following are the built-in features of the PythonQt library:
- Access all slots, properties, children and registered enums of any QObject derived class from Python
- Connecting Qt Signals to Python functions (both from within Python and from C++)
- Easy wrapping of Python objects from C++ with smart, reference-counting PythonQtObjectPtr.
- Convenient conversions to/from QVariant for PythonQtObjectPtr.
- Wrapping of C++ objects (which are not derived from QObject) via PythonQtCppWrapperFactory
- Extending C++ and QObject derived classes with additional slots, static methods and constructors (see Decorators)
- StdOut/Err redirection to Qt signals instead of cout
- Interface for creating your own
import
replacement, so that Python scripts can be e.g. signed/verified before they are executed (PythonQtImportFileInterface) - Mapping of plain-old-datatypes and ALL QVariant types to and from Python
- Support for wrapping of user QVariant types which are registerd via QMetaType
- Support for Qt namespace (with all enumerators)
- All PythonQt wrapped objects support the dir() statement, so that you can see easily which attributes a QObject, CPP object or QVariant has
- No preprocessing/wrapping tool needs to be started, PythonQt can script any QObject without prior knowledge about it (except for the MetaObject information from the moc)
- Multiple inheritance for C++ objects (e.g. a QWidget is derived from QObject and QPaintDevice, PythonQt will automatically cast a QWidget to a QPaintDevice when needed)
- Polymorphic downcasting (if e.g. PythonQt sees a QEvent, it can downcast it depending on the type(), so the Python e.g. sees a QPaintEvent instead of a plain QEvent)
- Deriving C++ objects from Python and overwriting virtual method with a Python implementation (requires usage of wrapper generator or manual work!)
- Extensible handler for Python/C++ conversion of complex types, e.g. mapping of QVector<SomeObject> to/from a Python array
- Setting of dynamic QObject properties via setProperty(), dynamic properties can be accessed for reading and writing like normal Python attributes (but creating a new property needs to be done with setProperty(), to distinguish from normal Python attributes)
- Support for QtCore.Signal, QtCore.Slot and QtCore.Property, including the creation of a dynamic QMetaObject.
PythonQt offers the additional PythonQt_QtAll library which wraps the complete Qt API, including all C++ classes and all non-slots on QObject derived classes. This offers the following features:
- Complete Qt API wrapped and accessible
- The following modules are available as submodules of the PythonQt module:
- QtCore
- QtGui
- QtNetwork
- QtOpenGL
- QtSql
- QtSvg
- QtWebKit
- QtXml
- QtXmlPatterns
- QtMultimedia
- QtQml
- QtQuick
- Any Qt class that has virtual methods can be easily derived from Python and the virtual methods can be reimplemented in Python
- Polymorphic downcasting on QEvent, QGraphicsItem, QStyleOption, ...
- Multiple inheritance support (e.g., QGraphicsTextItem is a QObject and a QGraphicsItem, PythonQt will handle this well)
- QtQuick support is experimental and currently it is not possible to register new qml components from Python
PythonQt supports:
Class Property Python
- Python 2 (>= Python 2.6)
- Python 3 (>= Python 3.3)
- Qt 4.x (Qt 4.7 and Qt 4.8 recommended)
- Qt 5.x (Tested with Qt 5.0, 5.3, 5.4 and 5.6)
Python Set Property
The last working Qt4 version is available at svn branches/Qt4LastWorkingVersion or you can download the PythonQt 3.0 release. The current svn trunk no longer supports Qt4, since we started to make use of some Qt5-only features.
Python Slots Property Search
- PythonQt is not as pythonic as PySide in many details (e.g. buffer protocol, pickling, translation support, ...) and it is mainly thought for embedding and intercommunication between Qt/Cpp and Python
- PythonQt offers properties as Python attributes, while PySide offers them as setter/getter methods (e.g. QWidget.width is a property in PythonQt and a method in PySide)
- PythonQt currently does not support instanceof checks for Qt classes, except for the exact match and derived Python classes
- QObject.emit to emit Qt signals from Python is not yet implemented but PythonQt allows to just emit a signal by calling it like a normal slot
- Ownership handling of objects is not as complete as in PySide and PySide, especially in situations where the ownership is not clearly passed to C++ on the C++ API.
- QStrings are always converted to unicode Python objects, QByteArray always stays a QByteArray and can be converted using QByteArray.data()
- Qt methods that take an extra 'bool* ok' parameter can be called passing PythonQt.BoolResult as parameter. In PySide, a tuple is returned instead.
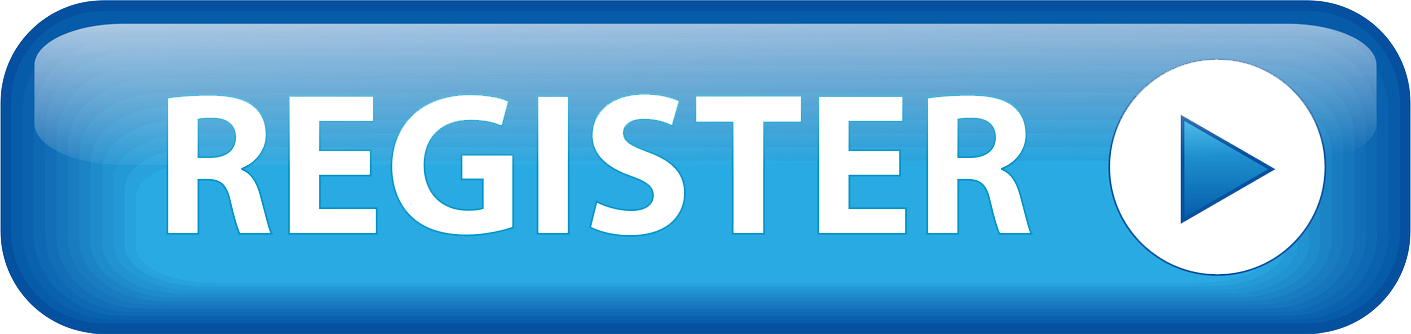